Is there a way to buy or transfer tokens from an ERC20 smart contract?
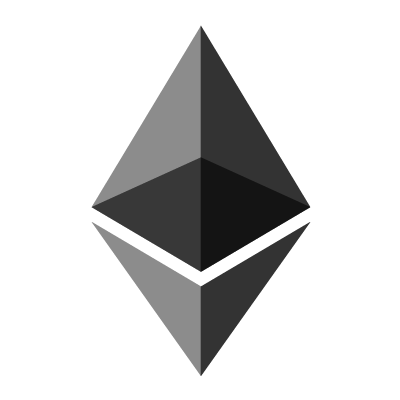
Hey everyone, I've been researching this question for months and all I was able to find anywhere is this:
Unfortunately I am fairly new to stack exchange and can't comment or ask a question. So I'm posting here incase someone has the same issue or wants to chime in.
This past summer, I followed a few blogs and video tutorials on creating an ERC20 standard token. I played with the code and tested it on the ropsten test net. Then finally deployed. Well, at the time I didn't know much about adding liquidity or listing on an exchange and was told that I need to send it to the smart contract in order for it to be bought. Now I feel that was a mistake and that they may be stuck.
Does anyone see anything in the code that would allow for it to be bought or transferred?
I thought this may be do-able in etherscan under "write contract" where there are options to "approve, transfer from, transfer", but that didn't seem to work.
Is there a way to transfer or buy the tokens from the smart contract to the contract creator?
I've pasted the contract code below:
pragma solidity ^0.5.0;
// —————————————————————————-
// ERC Token Standard #20 Interface
//
// —————————————————————————-
contract ERC20Interface {
function totalSupply() public view returns (uint);
function balanceOf(address tokenOwner) public view returns (uint balance);
function allowance(address tokenOwner, address spender) public view returns (uint remaining);
function transfer(address to, uint tokens) public returns (bool success);
function approve(address spender, uint tokens) public returns (bool success);
function transferFrom(address from, address to, uint tokens) public returns (bool success);
event Transfer(address indexed from, address indexed to, uint tokens);
event Approval(address indexed tokenOwner, address indexed spender, uint tokens);
}
// —————————————————————————-
// Safe Math Library
// —————————————————————————-
contract SafeMath {
function safeAdd(uint a, uint b) public pure returns (uint c) {
c = a + b;
require(c >= a);
}
function safeSub(uint a, uint b) public pure returns (uint c) {
require(b <= a); c = a – b; } function safeMul(uint a, uint b) public pure returns (uint c) { c = a * b; require(a == 0 || c / a == b); } function safeDiv(uint a, uint b) public pure returns (uint c) { require(b > 0);
c = a / b;
}
}
contract TokenExample is ERC20Interface, SafeMath {
string public name;
string public symbol;
uint8 public decimals; // 18 decimals is the strongly suggested default, avoid changing it
uint256 public _totalSupply;
mapping(address => uint) balances;
mapping(address => mapping(address => uint)) allowed;
/**
* Constrctor function
*
* Initializes contract with initial supply tokens to the creator of the contract
*/
constructor() public {
name = "TokenExample";
symbol = "TE";
decimals = 18;
_totalSupply = 12000000001000000000000000000;
balances[msg.sender] = _totalSupply;
emit Transfer(address(0), msg.sender, _totalSupply);
}
function totalSupply() public view returns (uint) {
return _totalSupply – balances[address(0)];
}
function balanceOf(address tokenOwner) public view returns (uint balance) {
return balances[tokenOwner];
}
function allowance(address tokenOwner, address spender) public view returns (uint remaining) {
return allowed[tokenOwner][spender];
}
function approve(address spender, uint tokens) public returns (bool success) {
allowed[msg.sender][spender] = tokens;
emit Approval(msg.sender, spender, tokens);
return true;
}
function transfer(address to, uint tokens) public returns (bool success) {
balances[msg.sender] = safeSub(balances[msg.sender], tokens);
balances[to] = safeAdd(balances[to], tokens);
emit Transfer(msg.sender, to, tokens);
return true;
}
function transferFrom(address from, address to, uint tokens) public returns (bool success) {
balances[from] = safeSub(balances[from], tokens);
allowed[from][msg.sender] = safeSub(allowed[from][msg.sender], tokens);
balances[to] = safeAdd(balances[to], tokens);
emit Transfer(from, to, tokens);
return true;
}
}
submitted by /u/ricksbongwater
[link] [comments]